Web Service is a software component for application to application communication.
There are two types of web services in Microsoft .Net framework. One is XML web services another one is .Net Remoting. In this article we are going to discuss about xml web service.
Web services are invoked remotely using SOAP or HTTP-GET and HTTP-POST protocols. Web service based on xml and returns the values in xml format.
Let we start our first web service…
Here I’m going to start my first web service as follows and named MyFirstService.
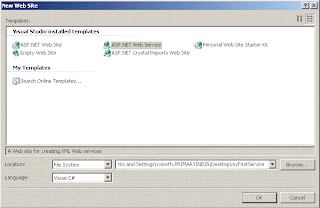
There is a .cs file in App_Code will be created. This is our class file for writing program.
Then a file Service.asmx will be created. This is our web service url.
Let me explain the simple web service method that returns an integer value with adding two integer values.
using System;
using System.Web;
using System.Web.Services;
using System.Web.Services.Protocols;
[WebService(Namespace = "http://tempuri.org/")]
[WebServiceBinding(ConformsTo = WsiProfiles.BasicProfile1_1)]
public class Service : System.Web.Services.WebService
{
public Service ()
{
}
[WebMethod]
public string HelloWorld() {
return "Hello World";
}
[WebMethod]
public int add(int a, int b)
{
return a + b;
}
}
Here the HelloWorld function is default function when we create the web service project.
The add function which is have two parameters (a & b) and return an integer value.
Running the program, and do as follows,
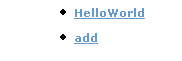
Click the add function…
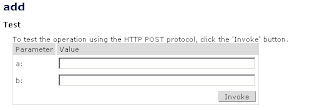
Here we should have to give the parameter.
Give the value as a=10 and b=10 and click the button Invoke.
After you will get the xml file as follows,
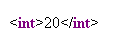
Hence we could create the service for adding two integer values.
CacheDuration attribute in Web Service
This attribute specifies the length of time in seconds that the method should cache the results.
For example,
[WebMethod(CacheDuration=30)] //Here 30 is seconds
public int add(int a, int b)
{
return a + b;
}
Description in WebMethod
We can add description in our web service’s WebMethod as follows,
[WebMethod(CacheDuration=30,Description="Returns added value.")] public int add(int a, int b)
{
return a + b;
}
We can see the description in the following picture marked in red.
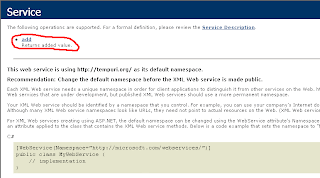
How to use webService in web application using C#
Yes..its a main thing how to use webservice in my application. Its very easy thing to use web service in our application by using AddWebReference. In the solution explorer we can find AddWebReference when we right click that solution explorer. Click that…
There is a window will open as follows,
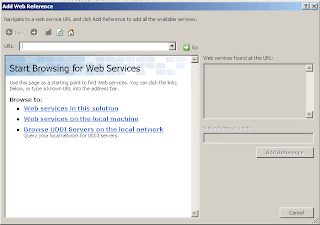
There is 3 options for adding our web reference.
- Web Services in this solution
Finding web service in your solution.
- Web services on the local machine
Finding web service in local machine or localhost.
- Browse UDDI Servers on the local network
Give your webservice url in that url column. And click Go…and give the web reference name as follows…
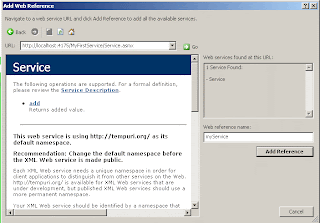
Then click the Add Reference button. Now see your solution explorer, there will be some new files with extensions .disco and .wsdl
Then put the namespace as
using myService;
And create object for that service then call the method in web service as follows,
Service service = new Service();
int result = service.add(10, 10);
this will return the value as 20.
That’s it…
...S.VinothkumaR.