Here is sample coding for how to empty the recycle bin using C#.
We can clear the recycle bin easily by using the Shell32.dll as follows,
First of all we need to create an enum as follows,
We can clear the recycle bin easily by using the Shell32.dll as follows,
First of all we need to create an enum as follows,
enum RecycleFlags : uint
{
SHERB_NOCONFIRMATION = 0x00000001,
SHERB_NOPROGRESSUI = 0x00000002,
SHERB_NOSOUND = 0x00000004
}
{
SHERB_NOCONFIRMATION = 0x00000001,
SHERB_NOPROGRESSUI = 0x00000002,
SHERB_NOSOUND = 0x00000004
}
And then, import the Shell32.dll using the DllImport class which is in System.Runtime.InteropServices namespaces. And creating the functionality SHEmptyRecycleBin as follows,
[DllImport("Shell32.dll",CharSet=CharSet.Unicode)]
static extern uint SHEmptyRecycleBin(IntPtr hwnd, string pszRootPath, RecycleFlags dwFlags);
static extern uint SHEmptyRecycleBin(IntPtr hwnd, string pszRootPath, RecycleFlags dwFlags);
In my project I have two buttons like “Clear the RecycleBin” and “Exit”.
When clicking the Clear the RecycleBin, we have to clear the recyclebin. So the following code will help for do that function. Copy the following code in to that button clicking event.
When clicking the Clear the RecycleBin, we have to clear the recyclebin. So the following code will help for do that function. Copy the following code in to that button clicking event.
try
{
uint result = SHEmptyRecycleBin(IntPtr.Zero, null, 0);
MessageBox.Show(this,"Done !","Empty the
RecycleBin",MessageBoxButtons.OK,MessageBoxIcon.Information);
}
catch(Exception ex)
{
MessageBox.Show(this, "Failed ! " + ex.Message, "Empty the
RecycleBin", MessageBoxButtons.OK, MessageBoxIcon.Stop);
Application.Exit();
}
{
uint result = SHEmptyRecycleBin(IntPtr.Zero, null, 0);
MessageBox.Show(this,"Done !","Empty the
RecycleBin",MessageBoxButtons.OK,MessageBoxIcon.Information);
}
catch(Exception ex)
{
MessageBox.Show(this, "Failed ! " + ex.Message, "Empty the
RecycleBin", MessageBoxButtons.OK, MessageBoxIcon.Stop);
Application.Exit();
}
Exit the application when click the “Exit” button.
Application.Exit();
Now run the program,
Application.Exit();
Now run the program,
Click the “Clear the Recycle Bin”
The confirmation window will appear for deleting all items for delete all items in the Recycle Bin.
Give yes to delete all items from Recycle Bin.
Give yes to delete all items from Recycle Bin.
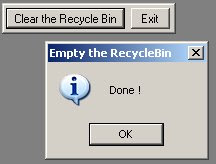
Yes…that’s it...
Cheers...
...S.VinothkumaR.
1 comment:
Hi...
Please take a look at following link.
http://www.mindstick.com/Blog/188/Recycle%20bin%20in%20c
This might be useful for you.
At this blog I will show you that how to work with recycle bin using c# such as emptying, retrieving file size and retrieving file numbers etc.
Post a Comment