Getting Drive Volume Information in C#
Just copy and paste the following code then run it...that's it. :)
using System.Runtime.InteropServices;
[DllImport("kernel32.dll", CharSet = CharSet.Auto)]
static extern bool GetVolumeInformation(string Volume,
StringBuilder VolumeName, uint VolumeNameSize,
out uint SerialNumber, out uint SerialNumberLength, out uint flags,
StringBuilder fs, uint fs_size);
private void Form1_Load(object sender, EventArgs e)
{
uint serialNum, serialNumLength, flags;
StringBuilder volumename = new StringBuilder(256);
StringBuilder fstype = new StringBuilder(256);
bool ok = false;
Cursor.Current = Cursors.WaitCursor;
foreach (string drives in Environment.GetLogicalDrives())
{
ok = GetVolumeInformation(drives, volumename, (uint)volumename.Capacity - 1, out serialNum,
out serialNumLength, out flags, fstype, (uint)fstype.Capacity - 1);
if (ok)
{
lblVolume.Text = lblVolume.Text + "\n Volume Information of " + drives + "\n";
lblVolume.Text = lblVolume.Text + "\nSerialNumber of is..... " + serialNum.ToString() + " \n";
if (volumename != null)
{
lblVolume.Text = lblVolume.Text + "VolumeName is..... " + volumename.ToString() + " \n";
}
if (fstype != null)
{
lblVolume.Text = lblVolume.Text + "FileType is..... " + fstype.ToString() + " \n";
}
}
ok = false;
}
Cursor.Current = Cursors.Default;
}
Hope this will helpful to you....
...S.VinothkumaR.
Nokia Secret Codes
*#06# for checking the IMEI (International Mobile Equipment Identity).
*#7780# reset to factory settings.
*#67705646# This will clear the LCD display(operator logo).
*#0000# To view software version.
*#2820# Bluetooth device address.
*#746025625# Sim clock allowed status.
#pw+1234567890+1# Shows if sim have restrictions.
*#92702689# - takes you to a secret menu where you may find some of the information below:
1. Displays Serial Number.
2. Displays the Month and Year of Manufacture
3. Displays (if there) the date where the phone was purchased (MMYY)
4. Displays the date of the last repair - if found (0000)
5. Shows life timer of phone (time passes since last start)
*#3370# - Enhanced Full Rate Codec (EFR) activation. Increase signal strength, better signal reception. It also help if u want to use GPRS and the service is not responding or too slow. Phone battery will drain faster though.
*#3370* - (EFR) deactivation. Phone will automatically restart. Increase battery life by 30% because phone receives less signal from network.
*#4720# - Half Rate Codec activation.
*#4720* - Half Rate Codec deactivation. The phone will automatically restart
If you forgot wallet code for Nokia S60 phone, use this code reset: *#7370925538#
Note, your data in the wallet will be erased. Phone will ask you the lock code. Default lock code is: 12345
Press *#3925538# to delete the contents and code of wallet.
Unlock service provider: Insert sim, turn phone on and press vol up(arrow keys) for 3 seconds, should say pin code. Press C,then press * message should flash, press * again and 04*pin*pin*pin# \
*#7328748263373738# resets security code.
Default security code is 12345
Change closed caller group (settings >security settings>user groups) to 00000 and ure phone will sound the message tone when you are near a radar speed trap. Setting it to 500 will cause your phone 2 set off security alarms at shop exits, gr8 for practical jokes! (works with some of the Nokia phones.) Press and hold "0" on the main screen to open wap browser.
...S.VinothkumaR.
Getting Disk Space using Management.
RadioButton rdDrives;
private void Form1_Load(object sender, EventArgs e)
{
int i = 10;
foreach (string drives in Environment.GetLogicalDrives())
{
rdDrives = new RadioButton();
rdDrives.Text = drives;
rdDrives.Top = i+20;
this.Controls.Add(rdDrives);
Application.DoEvents();
i = i + 40;
}
}
private void btnGetDiskSpace_Click(object sender, EventArgs e)
{
string drive = "";
RadioButton rd;
//Control[] control = this.Controls.Find("RadioButton", false);
for (int j = 0; j < rd =" (RadioButton)this.Controls[j];" drive =" rd.Text;" drive =" drive.Substring(0,">
private void getSize(string drive)
{
ManagementObject diskSize = new ManagementObject("win32_logicaldisk.deviceid=\"" + drive + ":\"");
diskSize.Get();
lblSize.Text = "Disk Space in " + drive + " is :" + diskSize["Size"] + " bytes";
lblFreeSpace.Text = "Free space in " +drive +" is :" + diskSize["FreeSpace"] + " bytes";
}
Getting Disk Information using DriveInfo in C#
I'm going to display all disk's information (VolumeLable, File System, Total Space, FreeSpace ...etc). Here is the code...check it out.
private void Form1_Load(object sender, EventArgs e)
{
DriveInfo[] allDrives = DriveInfo.GetDrives();
Label lbl;
int i = 0;
foreach (DriveInfo d in allDrives)
{
lbl = new Label();
lbl.AutoSize = true;
lbl.Text = "Drive: " + d.Name.Substring(0,1) + "\n";
lbl.Text = lbl.Text + "FileType: " + d.DriveType + "\n";
if (d.IsReady)
{
lbl.Text = lbl.Text + "Volume label: " + d.VolumeLabel+ "\n";
lbl.Text = lbl.Text + "File system: " + d.DriveFormat + "\n";
lbl.Text = lbl.Text + "Available space to current user: " + d.AvailableFreeSpace + "bytes\n";
lbl.Text = lbl.Text + "Total available space: " + d.TotalFreeSpace + "\n";
lbl.Text = lbl.Text + "Total size of drive: " + d.TotalSize + "\n";
}
lbl.Top = i;
this.Controls.Add(lbl);
i = i + 110;
}
}
That's it...
...S.VinothkumaR.
Convert .wav to .mp3 using Lame.exe in C#
{
//maxLen is in ms (1000 = 1 second)
string outfile= "-b 32 --resample 22.05 -m m \""+pworkingDir+fileName + "\" \"" + pworkingDir+fileName.Replace(".wav",".mp3")+"\"";
System.Diagnostics.ProcessStartInfo psi=new System.Diagnostics.ProcessStartInfo();
psi.FileName="\""+pworkingDir+"lame.exe"+"\"";
psi.Arguments=outfile;
//psi.WorkingDirectory=pworkingDir;
psi.WindowStyle=System.Diagnostics.ProcessWindowStyle.Minimized;
System.Diagnostics.Process p=System.Diagnostics.Process.Start(psi);
if (waitFlag)
{
p.WaitForExit();
// wait for exit of called application
}
}
This code will help us to convert .wav file in to .mp3
...S.VinothkumaR.
Compress mp3 file using Lame.exe in C#
Code:
private void mp3Encode(string srcFileName, string targetFileName)
{
if (!string.IsNullOrEmpty(srcFileName) &&
!string.IsNullOrEmpty(targetFileName))
{
try
{
string workingDir = AppDomain.CurrentDomain.BaseDirectory + "\\";
string outfile = "-V9 -q0 --resample 32 -B56 --verbose " + '"' + srcFileName + "\" \"" +
targetFileName + "\"";
System.Diagnostics.ProcessStartInfo psi = new System.Diagnostics.ProcessStartInfo();
psi.FileName = workingDir + "lame.exe";
psi.Arguments = outfile;
psi.WindowStyle = System.Diagnostics.ProcessWindowStyle.Hidden;
p = System.Diagnostics.Process.Start(psi);
while (p.HasExited == false)
{
Thread.Sleep(10);
Application.DoEvents();
}
p.Dispose();
}
catch (Exception ex)
{
MessageBox.Show(ex.Message);
}
}
}
private void frmConvertor_FormClosing(object sender, FormClosingEventArgs e)
{
if (p != null)
{
p.Dispose();
}
}
Hope this will help us to reduce our mp3 file’s size…
....S.VinothkumaR.
mciSendString in C#
Code:
using System.Runtime.InteropServices;
[DllImport("winmm.dll")]
private static extern long mciSendString(string strCommand, StringBuilder strReturn, int iReturnLength, IntPtr oCallback);
public string playCommand;
public string fileName;
public bool isPlay = false;
public bool isStop = false;
private void frmPlayer_Load(object sender, EventArgs e)
{
if (!string.IsNullOrEmpty(fileName))
{
try
{
if (File.Exists(fileName))
{
if (isPlay)
{
playCommand = "Close MediaFile";
mciSendString(playCommand, null, 0, IntPtr.Zero);
isPlay = false;
isStop = true;
}
playCommand = "open \"" + fileName + "\" type mpegvideo alias MediaFile";
mciSendString(playCommand, null, 0, IntPtr.Zero);
playCommand = "play MediaFile";
mciSendString(playCommand, null, 0, IntPtr.Zero);
isPlay = true;
btnPlayPlayer.Enabled = true;
btnPause.Enabled = true;
btnStop.Enabled = true;
}
else
{
isPlay = false;
btnPlayPlayer.Enabled = false;
btnPause.Enabled = false;
btnStop.Enabled = false;
MessageBox.Show("Invalid File!");
}
}
catch (Exception ex)
{
isPlay = false;
btnPlayPlayer.Enabled = false;
btnPause.Enabled = false;
btnStop.Enabled = false;
MessageBox.Show(ex.Message);
}
}
}
private void btnPlayPlayer_Click(object sender, EventArgs e)
{
if (!isPlay)
{
if (isStop)
{
playCommand = "open \"" + fileName + "\" type mpegvideo alias MediaFile";
mciSendString(playCommand, null, 0, IntPtr.Zero);
playCommand = "play MediaFile";
mciSendString(playCommand, null, 0, IntPtr.Zero);
isPlay = true;
isStop = false;
}
else
{
playCommand = "play MediaFile";
mciSendString(playCommand, null, 0, IntPtr.Zero);
isPlay = true;
}
}
}
private void btnPause_Click(object sender, EventArgs e)
{
if (isPlay)
{
playCommand = "pause MediaFile";
mciSendString(playCommand, null, 0, IntPtr.Zero);
isPlay = false;
}
}
private void btnStop_Click(object sender, EventArgs e)
{
if (isPlay)
{
playCommand = "Close MediaFile";
mciSendString(playCommand, null, 0, IntPtr.Zero);
isPlay = false;
isStop = true;
}
}
This coding will help us to play media file…
....S.VinothkumaR.
Launch Configuration for Android application in Eclipse
To create a launch configuration for the application, follow these steps:
1. Select Run > Open Run Dialog... or Run > Open Debug Dialog... as appropriate.
2. In the project type list on the left, right-click Android Application and select New.
3. Enter a name for your configuration.
4. On the Android tab, browse for the project and Activity to start.
5. On the Emulator tab, set the desired screen and network properties
6. You can set additional options on the Common tab as desired.
7. Press Apply to save the launch configuration, or press Run or Debug (as appropriate).
....S.VinothkumaR.
Creating an Android application in Eclipse
1. Select File > New > Project
2. Select Android > Android Project, and press Next
3. Select the contents for the project:
- Select Create new project in workspace to start a project for new code.
Enter the project name, the base package name, the name of a single Activity class to create as a stub .java file, and a name to use for your application.
- Select Create project from existing source to start a project from existing code. Use this option if you want to build and run any of the sample applications included with the SDK. The sample applications are located in the samples/ directory in the SDK.
Browse to the directory containing the existing source code and click OK. If the directory contains a valid Android manifest file, the ADT plugin fills in the package, activity, and application names for you.
4. Press Finish.
The ADT plugin creates the these folders and files for you as appropriate for the type of project:
- src/ A folder that includes your stub .java Activity file.
- res/ A folder for your resources.
- AndroidManifest.xml The manifest for your project.
.....S.VinothkumaR.
Download and Install Android SDK
We can develop Android application using eclipse IDE. For that we have to update the eclipse plugin as follows.
1. Start Eclipse, then select Help > Software Updates > Find and Install....
2. In the dialog that appears, select Search for new features to install and press Next.
3. Press New Remote Site.
4. In the resulting dialog box, enter a name for the remote site (e.g. Android Plugin) and enter this as its URL:
https://dl-ssl.google.com/android/eclipse/
Press OK.
5. You should now see the new site added to the search list (and checked). Press Finish.
6. In the subsequent Search Results dialog box, select the checkbox for Android Plugin > Eclipse Integration > Android Development Tools and press Next.
7. Read the license agreement and then select Accept terms of the license agreement, if appropriate. Press Next.
8. Press Finish.
9. The ADT plugin is not signed; you can accept the installation anyway by pressing Install All.
10. Restart Eclipse.
11. After restart, update your Eclipse preferences to point to the SDK directory:
a) Select Window > Preferences... to open the Preferences panel. (Mac OS X: Eclipse > Preferences)
b) Select Android from the left panel.
c) For the SDK Location in the main panel, press Browse... and locate the SDK directory.
d) Press Apply, then OK.
Updating the ADT Plugin
To update the ADT plugin to the latest version, follow these steps:
1. Select Help > Software Updates > Find and Install....
2. Select Search for updates of the currently installed features and press Finish.
3. If any update for ADT is available, select and install.
Alternatively,
1. Select Help > Software Updates > Manage Configuration.
2. Navigate down the tree and select Android Development Tools
3. Select Scan for Updates under Available Tasks.
That's it...
...S.VinothkumaR.
Android
If you want to know how to develop applications for Android, you can visit the google android page...
Image Uploading in ASP.NET using C#
Here is I’m going to post image in to an url with image byte array. On there a page will save that image byte array in to image in given path.
POST IMAGE
As I mentioned above, the following method is going to use post image.
using System.Net;
using System.Text;
using System.IO;
using System.Drawing.Imaging;
using System.Drawing;
private void postImage()
{
HttpWebRequest request;
request = (HttpWebRequest)HttpWebRequest.Create("url");
request.KeepAlive = true;
request.Method = "POST";
byte[] byteArray = ConvertImageToByteArray((System.Drawing.Image)new Bitmap(@"C:\test.jpg"), ImageFormat.Jpeg);
request.ContentType = "image/JPEG";
request.ContentLength = byteArray.Length;
Stream newStream = request.GetRequestStream();
newStream.Write(byteArray, 0, byteArray.Length);
newStream.Close();
}
private static byte[] ConvertImageToByteArray(System.Drawing.Image imageToConvert,ImageFormat formatOfImage)
{
byte[] Ret;
try
{
using (MemoryStream ms = new MemoryStream())
{
imageToConvert.Save(ms, formatOfImage);
Ret = ms.ToArray();
}
}
catch (Exception) { throw; }
return Ret;
}
From above the method postImage() will post the image in to given url as byte array. And the method ConvertImageToByteArray(System.Drawing.Image imageToConvert,ImageFormat formatOfImage) will help us for getting byte array from an image.
UPLOAD IMAGE
The following code will help us save the image….
using System.IO;
using System.Drawing;
using System.Drawing.Imaging;
using System.Net;
using System.Text;
protected void Page_Load(object sender, EventArgs e)
{
try
{
string dirPath = Server.MapPath("Asset") + "\\" ;
Guid imgId = Guid.NewGuid();
string imgPath = dirPath + "\\" + imgId.ToString() + ".jpg";
Response.ContentType = "image/JPEG";
System.Drawing.Image img = Bitmap.FromStream(Page.Request.InputStream);
img.Save(imgPath, ImageFormat.Jpeg);
}
catch { }
}
That's it....
...S.VinothkumaR.
Getting byte array from an image.
{
byte[] Ret;
try
{
using (MemoryStream ms = new MemoryStream())
{
imageToConvert.Save(ms, formatOfImage);
Ret = ms.ToArray();
}
}
catch (Exception) { throw; }
return Ret;
}
...S.VinothkumaR.
Boxing And UnBoxing
Now let us get an idea regarding reference type and value type objects. We all know that a new operator returns the memory address of an object that is allocated from the managed memory (a pool of memory). We usually store this address in a variable for our convenience. These types of variables are known as reference variables. However, the value type variables are not supposed to have the references; they always used to have the object itself and not the reference to it.
Now if we consider the statement written above in Listing 3, the C# compiler will detect System.Int32 as a value type and the object is not allocated from the memory heap, assuming this as an unmanaged one.
In a general way we should declare a type as a value type if the following are true.
1. The type should be a primitive type.
2. The type does not need to be inherited from any other types available.
3. No other types should also be derived from it.
This example shows how an integer type is converted to a reference type using the boxing and then unboxed from the object type to the integer type.
class Test
{
static void Main()
{
int i = 1; // i is an integer. It is a value type variable.
object o = i;
// boxing is happening. The integer type is parsed to //object type
int j = (int)o;
// unboxing is happening. The object type is unboxed to //the value type
}
}
Leap Year checking in Javascript
Here is I'm going to check the given year is leap or not...How to do....check it out..
I don't state that this code is perfect but it does work.
Just copy and paste the following function in to script tag inside the header tag so that it can be called from anywhere in this HTML document.
function isleap()
{
var yr=document.getElementById("year").value;
if ((parseInt(yr)%4) == 0)
{
if (parseInt(yr)%100 == 0)
{
if (parseInt(yr)%400 != 0)
{
alert("Not Leap");
return "false";
}
if (parseInt(yr)%400 == 0)
{
alert("Leap");
return "true";
}
}
if (parseInt(yr)%100 != 0)
{
alert("Leap");
return "true";
}
}
if ((parseInt(yr)%4) != 0)
{
alert("Not Leap");
return "false";
}
}
Basically this code gives the rules to check if a year is a leap year. If the year is no divisible by 4 then it is not a leap year. If the year is divisible by 100 but not by 400 then it is not a leap year. Otherwise the remaining options leave us with a leap year so I hard coded what to do. The Reason I have coded in what to do otherwise is to highlight the two different statements of verifying that something does match and verifying something does not match.
Just call this script function where you want...as follows,
onclick="isleap()"
that's it....
...S.VinothkumaR.
Caching in ASP.NET 2.0
· Output Caching
· Fragment Caching
· Data Caching
· Cache Configuration
Output Caching
Performance is improved in an ASP.NET application by caching the rendered markup and serving the cached version of the markup until the cache expires. For example, if you have a page that displays user information from a database, caching will help improve performance by serving the page from memory instead of making a connection to the database on each page request.
You can cache a page by using the OutputCache API or simply by using the @OutputCache directive.
<%@ Page Language=”C#” %>
<%@ OutputCache Duration=”15” VaryByParam=”none” %>
The above cache directive will cache the page for 15 minutes.
So output caching is great for when you want to cache an entire page.
Fragment Caching
In many situations caching the entire page just isn’t going to cut it, for some reason or another you require specific sections of the page to display live information. One way to improve performance is to analyze your page and identify objects that require a substantial overhead to run. You can build a list of these objects that are expensive to run, and then cache them for a period of time using fragment caching.
For example, say your page default.aspx consists of three user controls. After looking over the code, you identified that you can cache one of them. You can simply add the caching directive to the top of the user control:
<%@ Control %>
<%@ OutputCache Duration=”5” VaryByParam=”none” %>
Now keep in mind that the actual page that contains the control is not cached, only the user control. This means that the default.aspx page will be rendered each and every page request, but the user control is only ‘run’ every 15 minutes.
Data Caching
So we know that we can cache an entire page, or a fragment of a page by caching down to the user control level. Wouldn’t it be great if we could cache down to the object level? The good news is you can with ASP.NET Data caching.
The cache consists of a dictionary collection that is private to each application in memory. To insert items in the cache simply provide the collection with a unique name:
Cache[“someKey”] = myObject;
Retrieving the object form the cache:
myObject = (MyObject)Cache[“someKey”];
It is a good time to point out that you should always remember to check for null, and be sure to caste to your datatype.
Cache Configuration
If you are familiar with how caching worked in ASP.NET 1.0, you realize that managing all the cache directions for all your pages could potentially get out of hand. ASP.NET 2.0 introduces Cache Profiles that helps you centrally manage your cache. Cache settings can be inherited by your pages, and overridden if required by using the OutputCache directive.
The page directive looks pretty much the same, expect this time it references a cache profile that you defined in your web.config file.
So each and every page that references the ‘myCacheProfile1’ can be centrally managed in the web.config, this means that any changes to the cache settings in the web.config file will be automatically changed on all your referenced pages.
...S.VinothkumaR.ASP.Net page life cycle
Here is I’m going to explain, behind the scenes when a ASP.NET page loads.
The following are the various stages a page goes through:
Page Request
The request page is either parsed or compiled, or fetched from the cache.
Start
Page properties like the Request and Response are set. The type of request is determined, specifically whether it is a new Request or it is a PostBack. Culturing properties are also determined via the pages ICulture property.
Page Initialization
All the controls on the given page are initialized with a UniqueID (please don’t confused this with the ID property as the UnqiueID is a unique, hierarchicial identifier which includes the server control’s naming container). Theming is also applied at this stage.
Load
If the current request is a PostBack, the data from the viewstate and control state is loaded to the appropriate properties of the controls.
Validation
The Validate method of all the validator controls are fired, which in turn sets the Boolean property IsValid of the controls.
Postback Event Handling
If the current request is a PostBack, all event handlers are called.
Rendering
ViewState data is saved for all the controls that have enabled viewstate. The Render method for all the controls is fired which writes its output to the OutputStream via a text writer.
Unload
Once the page has been rendered and sent, the Page’s properties are unloaded (cleanup time).
So know you have a better understanding of the various stages of a ASP.NET pages life cycle, you should be aware that within each of the above stages there are events that you can hook into so that your code is fired at the exact time that you want it to.
Event Wire-up is another important concept to understand. So Event wire-up is where ASP.NET looks for methods that match a naming convention (e.g. Page_Load, Page_Init, etc) , and these methods are automatically fired at the appropriate event. There is a page level attribute AutoEventWireup that can be set to either true or false to enable this behaviour.
Below are some of the more popular events that you should understand as you will most likely be interested in them:
PreInit
You can use this event to:
· Create /recreate dynamic controls
· Set a master page or theme dynamically
· Access profile properties
Init
Used to read or initialize your control properties.
InitComplete
Used when you need to access your properties after they have been initialized.
PreLoad
Used when you need to process things before the Load event has been fired.
Load
The Page’s OnLoad method and all of its child control’s OnLoad method are fired recursively. This event is used to set properties and make database connections
Control Events
Control specific events are handled at this stage. e.g. Click event’s for the button control.
LoadComplete
This stage is for when you need to access controls that have been properly loaded.
PreRender
Can be used to make any ‘last chance’ changes to the controls before they are rendered.
SaveStateComplete
This event is used for things that require view state to be saved, yet not making any changes to the controls themselves.
Render
The page object calls this method on all of the controls, so all the controls are written and sent to the browser.
Unload
All the controls UnLoad method are fired, followed by the pages UnLoad event (bottom-up). This stage is used for closing database connections, closing open files, etc.
It is import to understand that each server control has its very own life cycle, but they are fired recursively so things may not occur at the time you think they do (they occur in reverse order!). What this means is that some events fire from the bottom up like the Init event, while others load from the top-down like the Load event....S.VinothkumaR.
LogOut Effect in IE, FireFox and OPERA
Copy and paste the following script in to your head section and call the log_out() in button click.
function log_out()
{
bdy = document.getElementsByTagName('body');
bdy[0].style.filter = 'Alpha(opacity="30")';
bdy[0].style.MozOpacity = '0.3';
bdy[0].style.opacity = '0.3';
if (confirm('Are you sure? Do you want to logout?'))
{
return true;
}
else
{
bdy[0].style.filter = 'Alpha(opacity="100")';
bdy[0].style.MozOpacity = '1';
bdy[0].style.opacity = '1';
return false;
}
}
...S.VinothkumaR.
DataTable in C#
Here is the sample code for creating and assigning values in to a datatable and set to datagrid via coding….
DataTable dt = new DataTable();
dt.Columns.Add("S.No", typeof(string));
dt.Columns.Add("UserID",typeof(string));
dt.Columns.Add("FamilyId",typeof(string));
dt.Columns.Add("PhotoGalleryId",typeof(string));
dt.Columns.Add("FileName",typeof(string));
dt.Columns.Add("Status", typeof(string));
dt.Columns.Add("Success", typeof(string));
dt.Columns.Add("ImagePath", typeof(string));
command=new SqlCommand("select * from [Assets]",connection);
connection.Open();
SqlDataReader reader = command.ExecuteReader();
int i = 1;
while(reader.Read())
{
Dr=dt.NewRow();
Dr[0] = i.ToString();
Dr[1] = reader.GetValue(1);
Dr[2] = reader.GetValue(2);
Dr[3] = reader.GetValue(3);
Dr[4] = reader.GetValue(4);
Dr[5] = reader.GetValue(5);
Dr[6] = reader.GetValue(7);
Dr[7] = ConfigurationManager.AppSettings["OrgImgAnchor"];
dt.Rows.Add(Dr);
i += 1;
}
grdStatus.DataSource = dt;
grdStatus.DataBind();
connection.Close();
...S.VinothkumaR.
Blackberry Development using Netbeans
Please let me know if you find any errors or missing steps.
Installation and setup:
Step 1 - download and install Suns Java SDK
Step 2 - download and install Netbeans IDE
Step 3 - download and install Netbeans Mobility Pack
Step 4 - download and install RIMs Blackberry JDE
Step 5 - Launch Netbeans, from the toolbar select tools > java platform manager > add platform and select ‘custom java micro edition platform emulator’
Step 6 - Enter (or paste) Rims JDE home directory, for example: ‘C:\Program Files\Research In Motion\BlackBerry JDE 4.2.0′ as the Platform Home, then add ‘Platform Name’ (eg. Rim) and ‘Device Name’ (eg. Blackberry)
Step 7 - Select ‘Next’, From the ‘Bootstrap Libraries’ list, remove every item except ‘net_rim_api.jar’
Step 8 - Select ‘Next’, Ignore the ‘Sources’ pane but add the path to the Blackberry javadocs, for example: ‘C:\Program Files\Research In Motion\BlackBerry JDE 4.2.0\docs\api\’ then click ‘finish’
Step 9 - Restart Netbeans
Create a simple Blackberry Application:Step 1 - Create a new project by selecting ‘mobile application’ from the ‘mobile category’, uncheck the ‘Create Hello Midlet’ option
Step 2 - Select the Blackberry platform that you’ve just created above
Step 3 - Add this xml data to the build.xml file which is visible if you select the ‘Files’ pane
Step 4 - Next you need to create an .alx file which is a Blackberry ‘Application Loader’ xml file, in the ‘Files’ pane, right-click and select ‘new’ > ‘empty file’
Name the file the same as your application (eg. myApp.alx), and add this xml data [important - only use numerics in the version number, any letters can cause issues for OTA installing of the application when you’re done, for example do not use any ‘beta’ version notifiers like b1.0
Step 5 - You’re now ready to start writing your application, switch back to the ‘Project’ pane and create your application, native Blackberry apps extend the UiApplication class instead of Midlet so you’ll have cheat Netbeans by entering your main class as the Midlet: right click on your application in the project pane and select ‘Properties’, under ‘Application Descriptor’ > MIDLets enter the name of your class that extends UiApplication (ignore any warnings)
Step 6 - Right click on your project and select ‘Clean and Build’, once you know your application will build select ‘Debug’ from the same menu. Netbeans should spawn the ‘Rim Remote Debug Server’ and the Blackberry Simulator, all your System.out.println statements will appear in the Debug Server not in the Netbeans output pane.
that's it....
...S.VinothkumaR.
Regex and MatchCollection
using System.Text.RegularExpressions;
string mail = "test
MatchCollection mcMail = Regex.Matches(mail, @"\w+?@\w+?\.(comnetin)");
string phoneNo = "My Phone Number is 98393 94893";
MatchCollection mcPhoneNo = Regex.Matches(phoneNo, @"\d\d\d\d\d \d\d\d\d\d");
if(mcMail.Count>0)
mail = mcMail[0].ToString();
if(mcPhoneNo.Count>0)
phoneNo = mcPhoneNo[0].ToString();
If we have a string with multiple phone number like "My Phone Number is 98393 94893 other no is 93759 84595", we can use Multiple MatchCollection as follows,
string phoneNo = "My Phone Number is 98393 94893 other no is 93759 84595";
MatchCollection mcPhoneNo = Regex.Matches(phoneNo, @"\d\d\d\d\d \d\d\d\d\d");
if (mcPhoneNo.Count > 0)
{
phoneNo = "";
for (int i = 0; i < phoneno ="phoneNo+mcPhoneNo[i].ToString()+">
From the MatchCollection, there are some properties available. Here is explonation for some of that properties.
Success:
Indicates whether a match was found. You should test this value before processing the match.
Index
Indicates the position in the string where the match starts.
Length
Indicates the length of the substring that matches the pattern. Value Equals the substring that did match the pattern.
Groups
Returns a collection of Group objects.
...S.VinothkumaR.
Log File creation for web application
public string logStatus;
logStatus = " Created! \t:" + DateTime.Now.ToString("hh:mm:ss");
if (!File.Exists(logPath))
{
File.WriteAllText(logPath, logStatus);
logStatus = System.Environment.NewLine;
logAppending(logStatus);
}
else
{
logAppending(logStatus);
}
logAppending
---------------
private void logAppending(string allStatus)
{
StreamWriter sw = new StreamWriter(logPath, true);
sw.WriteLine(allStatus + System.Environment.NewLine);
sw.Close();
}
...S.VinothkumaR.
Getting Image from Panel
Rectangle rect = new Rectangle(0, 0, panel1.Width, panel1.Height);
panel1.DrawToBitmap(bmp, rect);
bmp.Save("test.jpg");
...S.VinothkumaR.
Converting List to byte[] in C#.
{
List
byte[] byteArr = Encoding.ASCII.GetBytes("Test");
lstByte.AddRange(byteArr);
return lstByte.ToArray();
}
...S.VinothkumaR.
Converting String to Stream in C#
...S.VinothkumaR.
String Encryption and Decryption in C# using Cryptography.Rijndael algorithm.
using System.Security.Cryptography;
using System.IO;
public static string Encrypt(string clearText, string Password)
{
byte[] clearBytes = System.Text.Encoding.Unicode.GetBytes(clearText);
PasswordDeriveBytes pdb = new PasswordDeriveBytes(Password,
new byte[] {0x49, 0x76, 0x61, 0x6e, 0x20, 0x4d,
0x65, 0x64, 0x76, 0x65, 0x64, 0x65, 0x76});
// PasswordDeriveBytes is for getting Key and IV.
// Using PasswordDeriveBytes object we are first getting 32 bytes for the Key (the default
//Rijndael key length is 256bit = 32bytes) and then 16 bytes for the IV.
// IV should always be the block size, which is by default 16 bytes (128 bit) for Rijndael.
byte[] encryptedData = Encrypt(clearBytes, pdb.GetBytes(32), pdb.GetBytes(16));
return Convert.ToBase64String(encryptedData);
}
// Encrypt a byte array into a byte array using a key and an IV
public static byte[] Encrypt(byte[] clearData, byte[] Key, byte[] IV)
{
MemoryStream ms = new MemoryStream();
Rijndael alg = Rijndael.Create();
// Algorithm. Rijndael is available on all platforms.
alg.Key = Key;
alg.IV = IV;
CryptoStream cs = new CryptoStream(ms, alg.CreateEncryptor(), CryptoStreamMode.Write);
//CryptoStream is for pumping our data.
cs.Write(clearData, 0, clearData.Length);
cs.Close();
byte[] encryptedData = ms.ToArray();
return encryptedData;
}
public static byte[] Decrypt(byte[] cipherData, byte[] Key, byte[] IV)
{
MemoryStream ms = new MemoryStream();
Rijndael alg = Rijndael.Create();
alg.Key = Key;
alg.IV = IV;
CryptoStream cs = new CryptoStream(ms,alg.CreateDecryptor(), CryptoStreamMode.Write);
cs.Write(cipherData, 0, cipherData.Length);
cs.Close();
byte[] decryptedData = ms.ToArray();
return decryptedData;
}
// Decrypt a string into a string using a password
// Uses Decrypt(byte[], byte[], byte[])
public static string Decrypt(string cipherText, string Password)
{
byte[] cipherBytes = Convert.FromBase64String(cipherText);
PasswordDeriveBytes pdb = new PasswordDeriveBytes(Password,
new byte[] {0x49, 0x76, 0x61, 0x6e, 0x20, 0x4d, 0x65,
0x64, 0x76, 0x65, 0x64, 0x65, 0x76});
byte[] decryptedData = Decrypt(cipherBytes, pdb.GetBytes(32), pdb.GetBytes(16));
return System.Text.Encoding.Unicode.GetString(decryptedData);
}
Play a mp3 sound using MediaPlayer in C#.
- First add the library from Com reference with the name of Windows Media Player (wmp.dll).
- Drag the media player control from tool box in to your form.
- Copy the following code into Form_Load
axWindowsMediaPlayer1.URL = "C:\\test.mp3";
axWindowsMediaPlayer1.Ctlcontrols.stop();
- Copy the following code when click the play button
axWindowsMediaPlayer1.Ctlcontrols.play();
- Copy the following code when click the pause button.
axWindowsMediaPlayer1.Ctlcontrols.pause();
- We can get the time duration of playing file as follows,
axWindowsMediaPlayer1.currentMedia.attributeCount.ToString();
axWindowsMediaPlayer1.currentMedia.durationString;
axWindowsMediaPlayer1.Ctlcontrols.currentPositionString;
...S.VinothkumaR.
Find All Text files in all directories.
private void Form1_Load(object sender, EventArgs e)
{
foreach (string drives in Environment.GetLogicalDrives()) // Getting Logical drivers
{
FindFiles(drives);
}
}
private void FindFiles(string drives)
{
try
{
string[] directory = Directory.GetDirectories(drives); // Getting directories from a Directory
if (directory.Length > 0)
{
for (int i = 0; i < directory.Length; i++)
{
try
{
string[] files = Directory.GetFiles(directory[i], "*.txt"); //Finding Text files only
if (files.Length > 0)
{
for (int j = 0; j < files.Length; j++)
{
try
{
lstAllFiles.Items.Add(files[j]); //Adding files in to a list box
}
catch { }
}
}
}
catch { }
FindFiles(directory[i]);
}
}
}
catch { }
}
Getting the Values of Controls on the Master Page
// gets a reference to a textbox control inside a contentplaceholder
ContentPlaceHolder mpContentPlaceHolder;
TextBox mpTextBox;
mpContentPlaceHolder = ( ContentPlaceHolder ) Master.FindControl("ContentPlaceHolder1");
if ( mpContentPlaceHolder != null )
{
mpTextBox = ( TextBox ) mpContentPlaceHolder.FindControl ( "TextBox1" );
if ( mpTextBox != null )
{ mpTextBox.Text = "TextBox found!"; }
}
RoleManagement in Asp.Net
DataSet.HasChanges using in C#.
private void UpdateDataSet(DataSet myDataSet)
{
// check for changes with the HasChanges method first.
if (!myDataSet.HasChanges(DataRowState.Modified))
return;
// create temporary DataSet variable.
DataSet tempDataSet;
// getChanges for modified rows only.
tempDataSet = myDataSet.GetChanges(DataRowState.Modified);
// check the DataSet for errors.
if (tempDataSet.HasErrors)
{
// ... insert code to resolve errors here ...
}
// after fixing errors, update db with the DataAdapter used to fill the DataSet.
myDataAdapter.Update(tempDataSet);
}
...S.VinothkumaR.
XML Serialization and DeSerialization using C#.
myClass.CS
using System;
using System.Collections.Generic;
using System.Text;
namespace Serialization
{
public class myClass
{
protected string namefield;
protected string placefield;
public string name
{
get { return namefield; }
set { namefield = value; }
}
public string place
{
get { return placefield; }
set { placefield = value; }
}
}
button1_Click
myClass myClassObj = new myClass();
myClassObj.name = "Vinoth";
myClassObj.place = "Chennai";
XmlSerializer serializer = new XmlSerializer(typeof(myClass));
string path = Environment.CurrentDirectory + "\\serialization.xml";
TextWriter writer = new StreamWriter(path,true);
serializer.Serialize(writer, myClassObj);
writer.Close();
MessageBox.Show("Serialized");
FileStream fs = File.OpenRead(Environment.CurrentDirectory + "\\serialization.xml");
myClassObj=(myClass)serializer.Deserialize(fs);
MessageBox.Show(myClassObj.name);
That's it....
....S.VinothkumaR.
XML to XSD And XSD to CS
For this we need to put our xml file in to c:\Program Files\Microsoft Visual Studio 8\VC and run the following command in visual studio command prompt.
xsd test.xml // here test.xml is our xml file.
The following command for creating a CSharp class from xsd.
xsd test.xsd /c
Converting a CS file in to DLL
First put your cs file in C:\Program Files\Microsoft Visual Studio 8\VC
And choose Dot Net command prompt as follows,
Start ->
All Programs ->
Microsoft Visual Studio 2005 ->
Visual Studio 2005 Command Prompt
Enter the following and press enter key,
csc /t:library /r:System.Web.Services.dll /r:System.Xml.dll myClass.cs
t --> means Target
r --> means Reference.
Note:
Here,
System.Web.Services.dll is webservice's reference.
System.Xml.dll is XML's reference.
Image Drag and Drop in WindowsApplication using C#.
----------------------------
AllowDrop Property set to True in which control you have to drag the image.
For Ex,
Here is I'm going to drag an image from a picture box in to a panel.
Step 1:
Set AllowDrop property to True for panel control.
Step 2:
In picturebox mouse down event, write the code as follows,
pictureBox1 = (PictureBox)sender;
DoDragDrop(pictureBox1.Image, DragDropEffects.Copy);
Step 3:
In panel Drag_DragEnter event, write the following,
if (e.Data.GetDataPresent(typeof(Bitmap)))
{
e.Effect = DragDropEffects.Copy;
}
else
{
e.Effect = DragDropEffects.None;
}
Step 4:
In panel Drag_DragDrop event, write the following,
Panel pnlDroggedTheme = (Panel)sender;
pnlDroggedTheme.Height = panel1.Height;
pnlDroggedTheme.Width = panel1.Width;
pnlDroggedTheme.BackgroundImage = (Bitmap)e.Data.GetData(typeof(Bitmap));
Run the program, that's it...
...S.VinothkumaR.
Images from DB in ASP.NET
public SqlConnection connection = new SqlConnection(ConfigurationManager.AppSettings(connectionstring);
public SqlCommand command;
protected void Button1_Click(object sender, EventArgs e)
{
command = new SqlCommand("select img_data from [tbl_image] where img_Id=1", connection);
connection.Open();
byte[] imgData= (byte[])this.command.ExecuteScalar();
MemoryStream memoryStream = new MemoryStream();
memoryStream.Write(imgData, 0, imgData.Length);
System.Drawing.Image img = System.Drawing.Image.FromStream(memoryStream);
Response.ContentType = "image/Jpeg";
img.Save(Response.OutputStream, System.Drawing.Imaging.ImageFormat.Jpeg);
connection.Close();
}
...S.VinothkumaR.
Image to ByteArray and ByteArray to Image using C#
----------------
private static byte[] GetImageByteArr(System.Drawing.Image img)
{
byte[] ImgByte;
using (MemoryStream stream = new MemoryStream())
{
img.Save(stream, System.Drawing.Imaging.ImageFormat.Jpeg);
ImgByte = stream.ToArray();
}
return ImgByte;
}
Byte[] to Image
----------------
private Image bytetoimg(byte[] bytearr)
{
Image newImage;
MemoryStream ms=new MemoryStream(bytearr,0,bytearr.Length);
ms.Write(bytearr,0,bytearr.Length);
newImage=Image.FromStream(ms,true);
return newImage;
}
...S.Vinothkumar
String to Byte Array And Byte Array to String.
Method 1
----------
public static byte[] StrToByteArray(string str)
{
System.Text.ASCIIEncoding encoding=new System.Text.ASCIIEncoding();
return encoding.GetBytes(str);
}
Method 2
----------
byte[] byteArray = Encoding.UTF8.GetBytes(stringValue);
Byte[] to String
----------------
byte [] dBytes = ...
string str;
System.Text.ASCIIEncoding enc = new System.Text.ASCIIEncoding();
str = enc.GetString(dBytes);
...S.VinothkumaR.
System Tray Icon using C#
Copy the following code in your form loading event.
private NotifyIcon appIcon = new NotifyIcon();
Icon ico = new Icon("icon.ico");
appIcon.Icon = ico;
appIcon.Text = "Vinoth Application";
appIcon.Visible = true;
put your icon file in your bin->Debug folder.
then run the application ...that's all.
How to list databases from DB?
string connectionString = "server=ServerName;uid=sa;pwd=pwd;";
using (SqlConnection connection = new SqlConnection(connectionString))
{
connection.Open();
DataTable dt= connection.GetSchema("Databases");
connection.Close();
foreach (DataRow row in dt.Rows)
{
Response.Output.WriteLine("Database: " + row["database_name"]);
}
}
WebClient Open Write using C#
using System;
using System.IO;
using System.Net;
public class myTest
{
WebClient webClient = new WebClient();
string data = "upload data using webclient.";
Stream stream = webClient .OpenWrite(url);
StreamWriter sw = new StreamWriter(stream);
sw.WriteLine(data);
sw.Close();
stream.Close();
}
HTTPWebRequest - POST Method.
HttpWebRequest request = (HttpWebRequest)HttpWebRequest.Create("url")
request.Method = "POST";
string parameter = "";
parameter += "test=test1&";
parameter += "testing=testing1&";
parameter += "demo=demo1";
byte[] byteArray = Encoding.UTF8.GetBytes(parameter);
request.ContentType = "application/x-www-form-urlencoded";
request.ContentLength = byteArray.Length;
Stream dataStream = request.GetRequestStream();
dataStream.Write(byteArray, 0, byteArray.Length);
dataStream.Close();
HttpWebResponse response = (HttpWebResponse)request.GetResponse();
if (HttpStatusCode.OK == response.StatusCode)
{
dataStream = response.GetResponseStream();
StreamReader reader = new StreamReader(dataStream);
responseFromServer = reader.ReadToEnd();
File.WriteAllText(filepath, responseFromServer);
response.Close();
}
HTTPWebRequest using PUT method.
try
{
string str = "test";
System.Text.ASCIIEncoding encoding = new System.Text.ASCIIEncoding();
byte[] arr = encoding.GetBytes(str);
HttpWebRequest request = (HttpWebRequest)HttpWebRequest.Create("url");
request.Method = "PUT";
request.ContentType = "text/plain";
request.ContentLength = arr.Length;
request.KeepAlive = true;
Stream dataStream = request.GetRequestStream();
dataStream.Write(arr, 0, arr.Length);
dataStream.Close();
HttpWebResponse response = (HttpWebResponse)request.GetResponse();
string returnString = response.StatusCode.ToString();
}
catch {}
Email sending - ASP.NET
try
{
SmtpClient client = new SmtpClient();
client.Credentials = new NetworkCredential("username", "password");//Here is your mail server's any username and password.
client.Host = "smtp.test.com";// Your mail server's smtp host address.
client.Send("fromAddress@test.com", "itvinoth83@gmail.com", "mail by Vinoth", "Test Mail");
}
catch (SmtpException ex)
{
Response.Write("SendEMail: " + ex.Message);
}
WebService using C#
Web Service is a software component for application to application communication.
There are two types of web services in Microsoft .Net framework. One is XML web services another one is .Net Remoting. In this article we are going to discuss about xml web service.
Web services are invoked remotely using SOAP or HTTP-GET and HTTP-POST protocols. Web service based on xml and returns the values in xml format.
Let we start our first web service…
Here I’m going to start my first web service as follows and named MyFirstService.
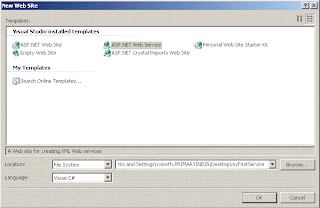
There is a .cs file in App_Code will be created. This is our class file for writing program.
Then a file Service.asmx will be created. This is our web service url.
Let me explain the simple web service method that returns an integer value with adding two integer values.
using System;
using System.Web;
using System.Web.Services;
using System.Web.Services.Protocols;
[WebService(Namespace = "http://tempuri.org/")]
[WebServiceBinding(ConformsTo = WsiProfiles.BasicProfile1_1)]
public class Service : System.Web.Services.WebService
{
public Service ()
{
}
[WebMethod]
public string HelloWorld() {
return "Hello World";
}
[WebMethod]
public int add(int a, int b)
{
return a + b;
}
}
Here the HelloWorld function is default function when we create the web service project.
The add function which is have two parameters (a & b) and return an integer value.
Running the program, and do as follows,
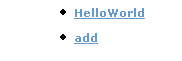
Click the add function…
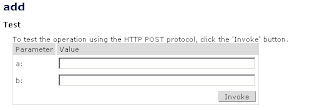
Here we should have to give the parameter.
Give the value as a=10 and b=10 and click the button Invoke.
After you will get the xml file as follows,
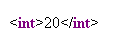
Hence we could create the service for adding two integer values.
CacheDuration attribute in Web Service
This attribute specifies the length of time in seconds that the method should cache the results.
For example,
[WebMethod(CacheDuration=30)] //Here 30 is seconds
public int add(int a, int b)
{
return a + b;
}
Description in WebMethod
We can add description in our web service’s WebMethod as follows,
[WebMethod(CacheDuration=30,Description="Returns added value.")] public int add(int a, int b)
{
return a + b;
}
We can see the description in the following picture marked in red.
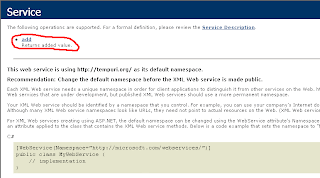
How to use webService in web application using C#
Yes..its a main thing how to use webservice in my application. Its very easy thing to use web service in our application by using AddWebReference. In the solution explorer we can find AddWebReference when we right click that solution explorer. Click that…
There is a window will open as follows,
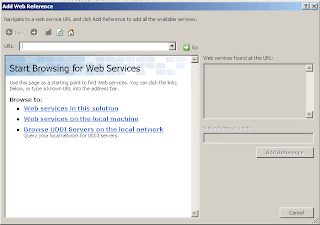
There is 3 options for adding our web reference.
- Web Services in this solution
Finding web service in your solution.
- Web services on the local machine
Finding web service in local machine or localhost.
- Browse UDDI Servers on the local network
Give your webservice url in that url column. And click Go…and give the web reference name as follows…
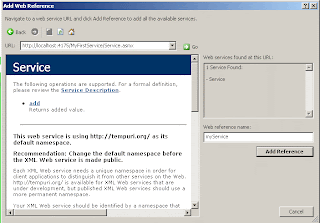
Then click the Add Reference button. Now see your solution explorer, there will be some new files with extensions .disco and .wsdl
Then put the namespace as
using myService;
And create object for that service then call the method in web service as follows,
Service service = new Service();
int result = service.add(10, 10);
this will return the value as 20.
That’s it…
...S.VinothkumaR.
Read And Write Registry Value using C#.
Here is the code for read the registry value using C#.
First I’m creating registry key value and string value in local machine as follows,
myKey --> key value
myValue --> string name
myReturnValue --> string value
Snapshots for registry values setting...



Code for Registry value Reading using C#...
using Microsoft.Win32;
try
{
RegistryKey registry = Registry.LocalMachine.CreateSubKey("SOFTWARE\\myKey");
if (registry != null)
{
MessageBox.Show(registry.GetValue("myValue"));
registry.Close();
}
}
catch (Exception ex)
{
MessageBox.Show (ex.ToString());
}
Code for Values writing in Registry using C#.
using Microsoft.Win32;
try
{
RegistryKey registry = Registry.LocalMachine.CreateSubKey("SOFTWARE\\myKey");
if (registry != null)
{
registry.SetValue("myValue", "myReturnValue");
registry.Close();
}
}
catch (Exception ex)
{
MessageBox.Show (ex.ToString());
}
... S.VinothkumaR.
CD Door Open and Close
Here is sample code for open the CD door and close using C#...
Just try...
using System.Runtime.InteropServices;
using System.Text;
[DllImport("winmm.dll", EntryPoint = "mciSendStringA", CharSet = CharSet.Ansi)]
protected static extern int mciSendString(string mciCommand, StringBuilder returnValue, int returnLength, IntPtr callback);
int result = mciSendString("set cdaudio door open", null, 0, IntPtr.Zero);
result = mciSendString("set cdaudio door closed", null, 0, IntPtr.Zero);
...S.VinothkumaR
Getting IP Address using C#
Here is I'm trying to get IP address in a web application when it's page load using C#. The following code will help u for getting IP address....
using System.Net;
string hostName = Dns.GetHostName();
IPHostEntry iPHostEntry = Dns.GetHostEntry(hostName);
IPAddress[] ipAddress = iPHostEntry.AddressList;
for (int i = 0; i < ipAddress.Length; i++)
{
Response.Write(ipAddress[i].ToString());
}
S.VinothkumaR
Empty the Recycle Bin using C#
We can clear the recycle bin easily by using the Shell32.dll as follows,
First of all we need to create an enum as follows,
{
SHERB_NOCONFIRMATION = 0x00000001,
SHERB_NOPROGRESSUI = 0x00000002,
SHERB_NOSOUND = 0x00000004
}
static extern uint SHEmptyRecycleBin(IntPtr hwnd, string pszRootPath, RecycleFlags dwFlags);
When clicking the Clear the RecycleBin, we have to clear the recyclebin. So the following code will help for do that function. Copy the following code in to that button clicking event.
{
uint result = SHEmptyRecycleBin(IntPtr.Zero, null, 0);
MessageBox.Show(this,"Done !","Empty the
RecycleBin",MessageBoxButtons.OK,MessageBoxIcon.Information);
}
catch(Exception ex)
{
MessageBox.Show(this, "Failed ! " + ex.Message, "Empty the
RecycleBin", MessageBoxButtons.OK, MessageBoxIcon.Stop);
Application.Exit();
}
Application.Exit();
Now run the program,
Give yes to delete all items from Recycle Bin.
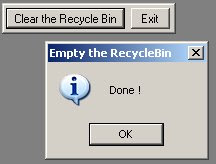
ASP.NET Menu using Sitemap
Creating Sitemap file.
The
- title (Specifies the title of the section).
- url (Specify the url of section. It must have unique).
- description (Specify the description of the section. Used in alt attribute).
Creating Master Page.
After completing this process go to master page and copy the following code

From the above, SiteMapDataSource is set to asp:Menu datasource. Then copy the following code in to sitemap file.

Now run the project …
That’s it…
If you want to download the program come here...
Cheers....
...VinothkumaR.